SuperFast Angular UX using Rx.js observables
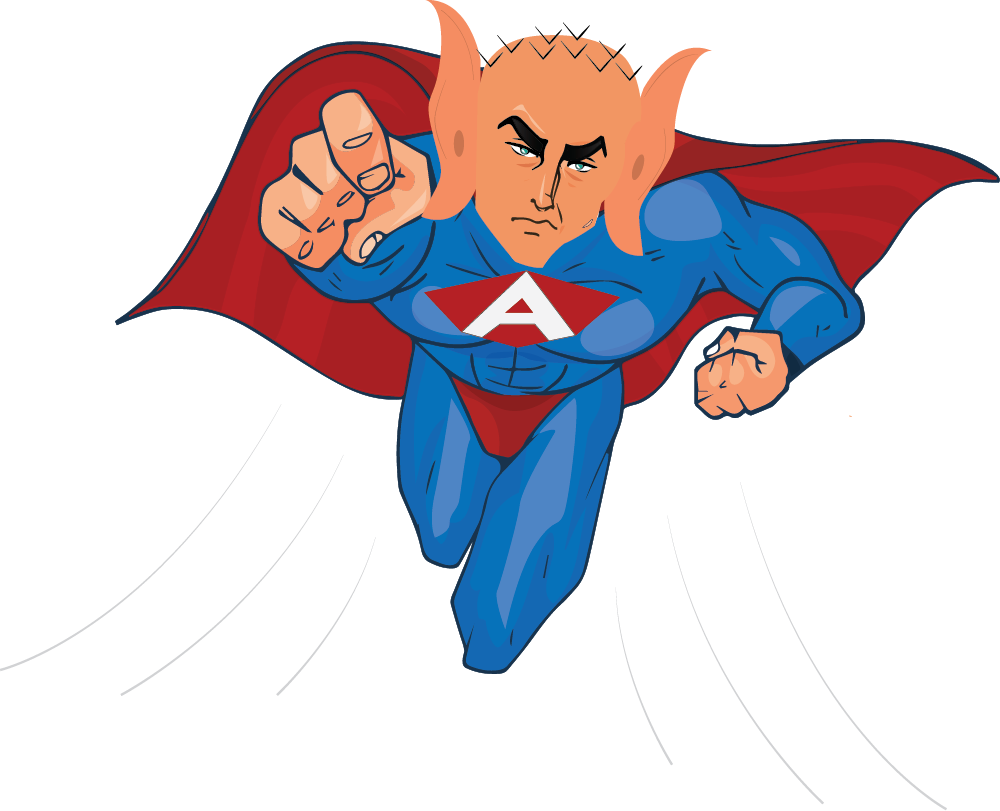
Today, web application (Web App) users are expecting blazing fast user experiences since the internet speed is no more a hurdle.
I am always a fan of keeping things super simple. However when I come across a lot of technologies and jargons like React, Angular, single page, big data, distributed architecture, Rx.js, Reactive architecture and … the list is endless, I get a little overwhelmed. I wonder if it is necessary to make the solutions so sophisticated.
The root cause
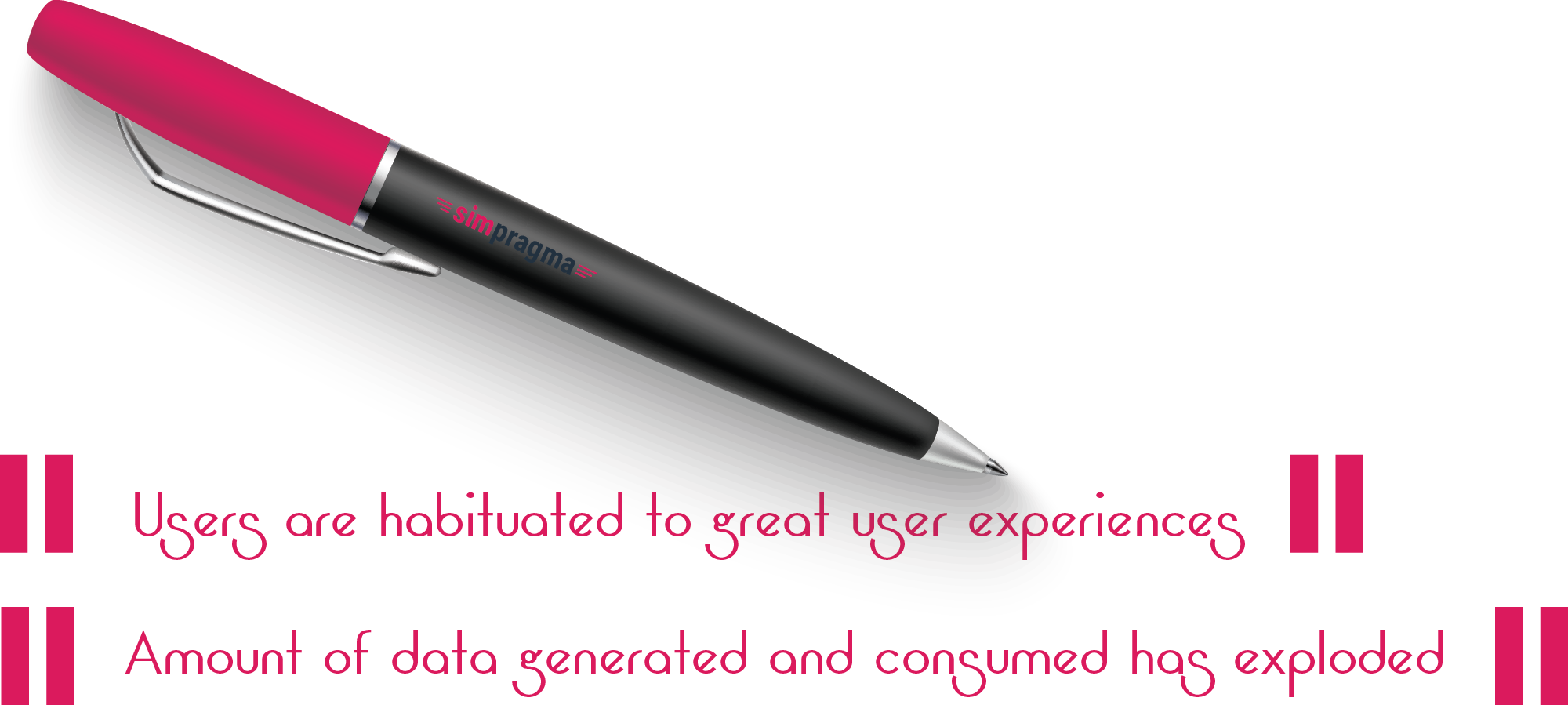
I see a clear wave of Digital transformation. The quantity of the data required to produce digital experiences is exploded. The speed at which the data has to be processed and presented is almost always supersonic.
Imagine, that we are collecting movement of people using RF ID trackers. We collect data every 15 seconds to track 1000 people, that would mean 5,760,000 readings every day. You can do the calculation of monthly and annual volumes.
Imagine keeping sensors to observe weather every 5 kilometres throughout a country like India
The Rx.js observable
The Rx.js observer fits the bill here. As such observer pattern is not new. It appeared in different shapes and forms in other technologies too. Rx.js is what both React and Angular employ. This pattern is now an essential element of every web app. This pattern will be part of the JavaScript language soon.
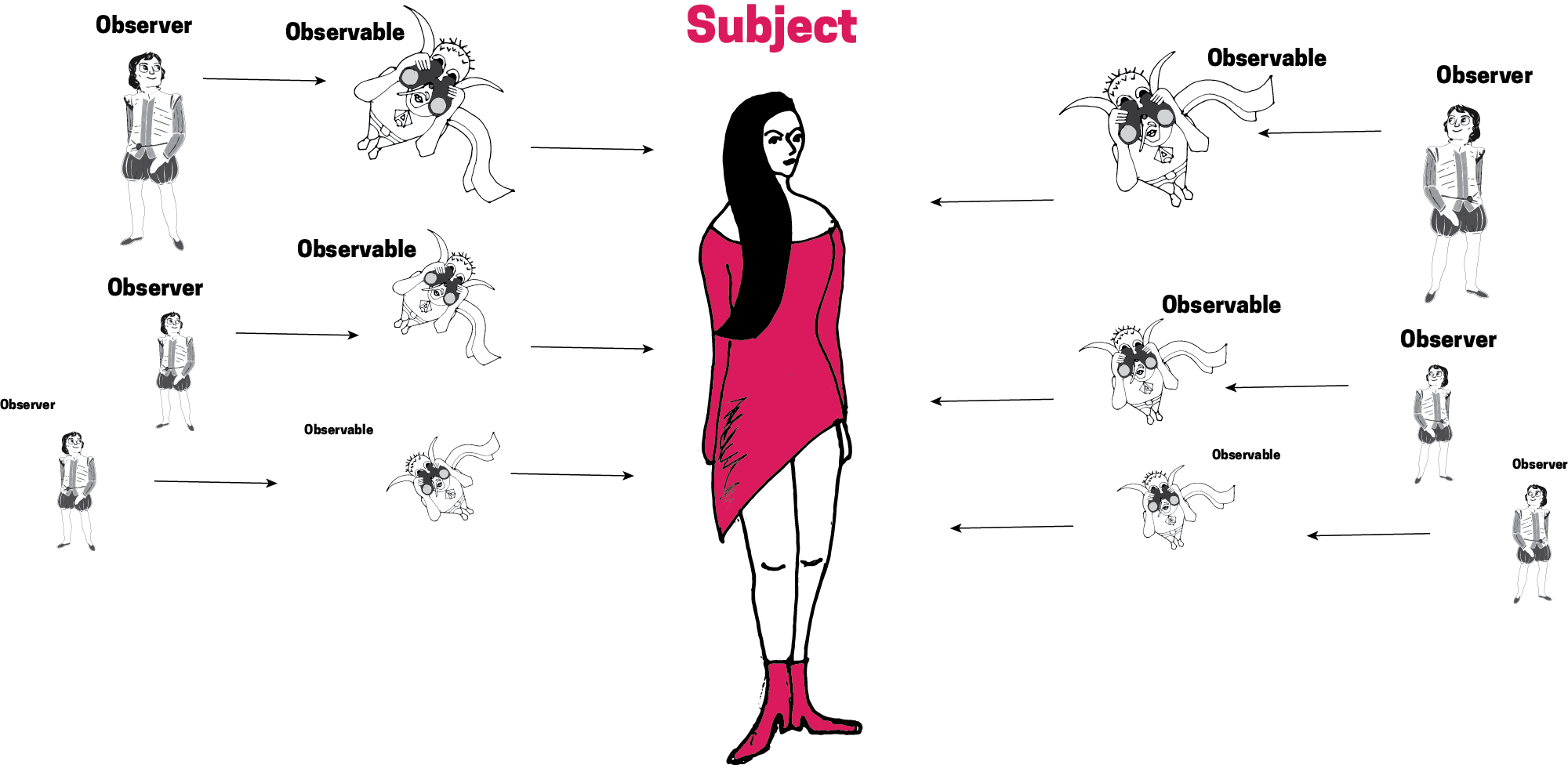
The observers designate an observable to watch a “Subject”.
Subject is any entity that is of interest to the web app. A lot like a pretty girl. Many Romeo’s would want to know which Yoga class she attends, what restaurant she visits, what blogs she reads and what music she likes. All the interested followers would like to know every activity and respond to it.
So they designate their observables who keep a watch and provide all the information in real time.
Let us consider some real life web app. An airline reservation Web App will continually want to know the rates and status of each flight. A blogging Web App would want to continuously update the new blogs that get produced. A news Web App is interested in every new news item. An Ecommerce Web App is interested in every new order.
It’s my life, it’s my pace.
However the world is full of varieties and options. So the “Subjects” are many. And, the observers can watch more than one subject. Every web app will need more than one subject. Every subject is needed by more than one web app.Every subject will produce events at its own pace. Ever observer will keep consuming at its own pace..
So for a web app, it is raining events everywhere, some from users and some from the subjects. All the updates should reflect on the user interface in an elegant way. There should be no disturbance to existing user interface, no sluggishness, no deterioration of the aesthetics, everything spick and span.
Method to the madness
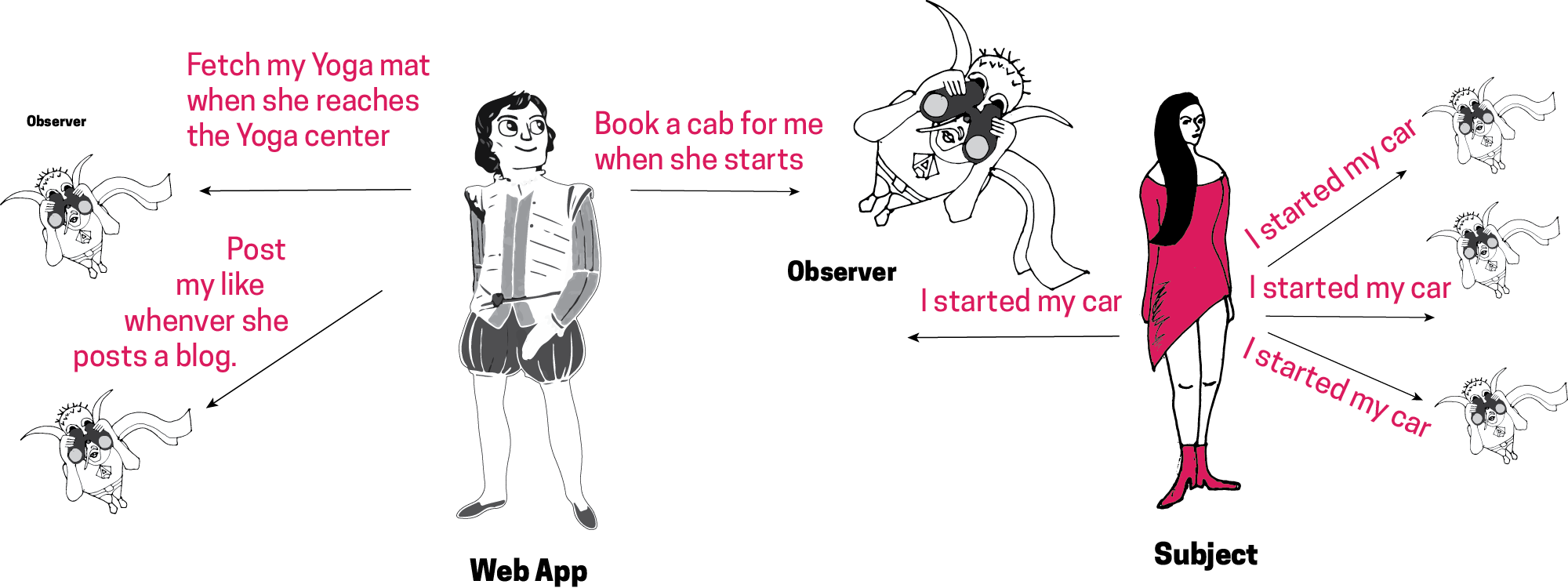
Here the madness is on both the sides. The subject caters to multiple applications. The applications consume updates from multiple subjects. So this sure calls for a well thought solution design. Here is how the whole traffic of updates is regulated.
Step 1:
The web app designates the observable to call some action when it receives updates from the subject. The web app subscribes the observable
Step 2:
The observable has to enter into a contract with subject to get the updates. This means that observable has to be registered with the subject.
Step 3:
The subject changes and updates all the contracted observables. One of the considerations here is to send the right set of updates to different observers.
Step 4:
The observable performs the actions specified by the Web App. These actions may include updating some objects or data structures inside the Web App.
Step 5:
Some components in the web app get changed due to the actions. The changed data structure of objects may affect multiple display components or user interfaces on the Web App.
Step 6:
These changes now have to be reflected on the user interfaces. Angular has a mechanism to update these changes automatically.Angular does in a way that makes minimal disturbance to the running application.
Step 7:
Angular provides support from cradle to grave. Wait a minute, we did not speak of grave yet. Yeah the step 7 would be to unsubscribe further updates.
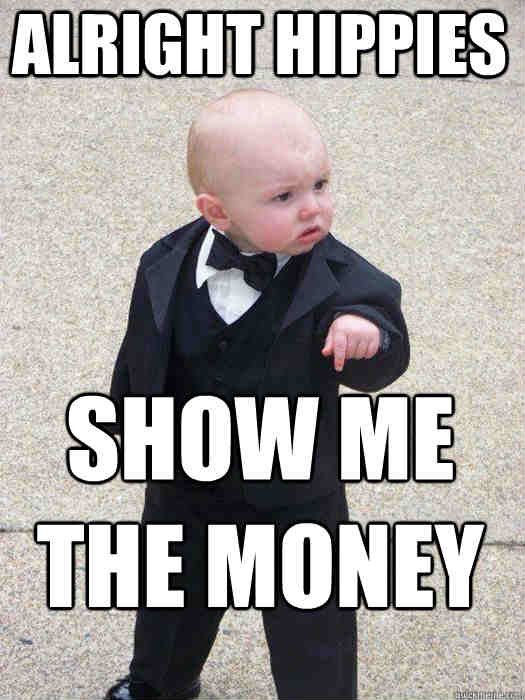
Done with speech, where is the action
That was all the concept of observables. I would also want to delve into Angular specific implementation.
The next section will cover an example in Angular that we will implement using observables.
A real life example using Angular
Let us develop a flight arrival dashboard for different cities. Since the API to get flight information is available, we make use of it. We make a quick list of our favourite airports and monitor the flight arrivals. Here is the demo - http://45.33.67.90:8081/observables-ui#/. Here is the source code for your reference - https://github.com/dilshad372/angular-observable/
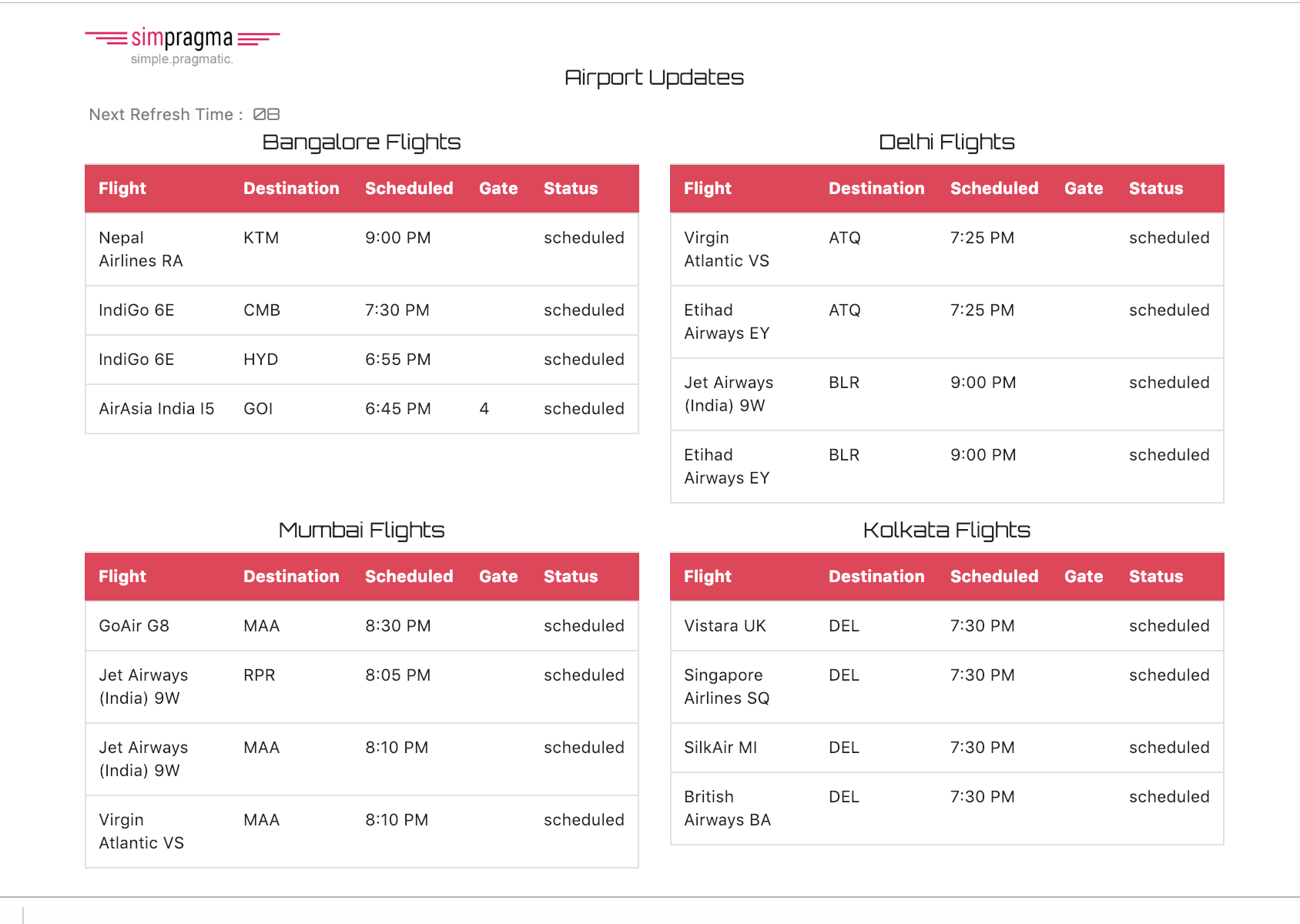
To understand the implementation, just to be on the same page I will mention some Angular concepts.Angular user interface is divided into multiple components to achieve modularity. Each component has its own view, logic and control. Each component will update itself independently on the user interface.
The main component
So we create our app a main component. The main component in turn will have four components. The four components are – Bangalore flights, Delhi flights, Chennai flights and Kolkata flights. The main component, all the services and all the subjects will be created during the app initiation. This means that the services will be running till the application is running. The subjects will be alive till the end of the application. The observers will be created and destroyed upon the creation and destruction of components. So will be the subscriptions.
The subjects
Rx.js provides “subjects” that have dual role. The role of an observer and the role of an observable. The observers are passed by the components to the subjects. The observers will contain the next function to be executed when the state of the subject changes. The services call the next function of the observables. This next function multicasts and calls the next function of all the observers. So we create four subjects corresponding to four user interfaces.
The services
For the four subjects, I have four services that in turn call RESTful API to get flight arrival details from airport details. The flight status service keeps polling the backend system using the provided REST API. Once the service receives an update it calls the method “next()” from the subject. The current mechanism we use here is "pull". However in case of event-driven programming "push" methods are more apt. We could use a message que or a websocket to get data in "push" way.
The flight arrival user interface components
Next we create the individual components for updating flight information for Bangalore, Kolkata, Chennai and Delhi. During initialisation of the components, the components subscribe to the corresponding subjects. To subscribe a subject, the component has to pass an observer. The observer will contain the function to be executed every time the subject changes. The subject registers this observer. When the service calls “next” function of subject, the subject in-turn calls the “next” function provided in each of the observers.
The next function of the observer updates an array within the component. Component updates the user interface automatically when in its next iteration of refreshing the component.
Here is the application flow:
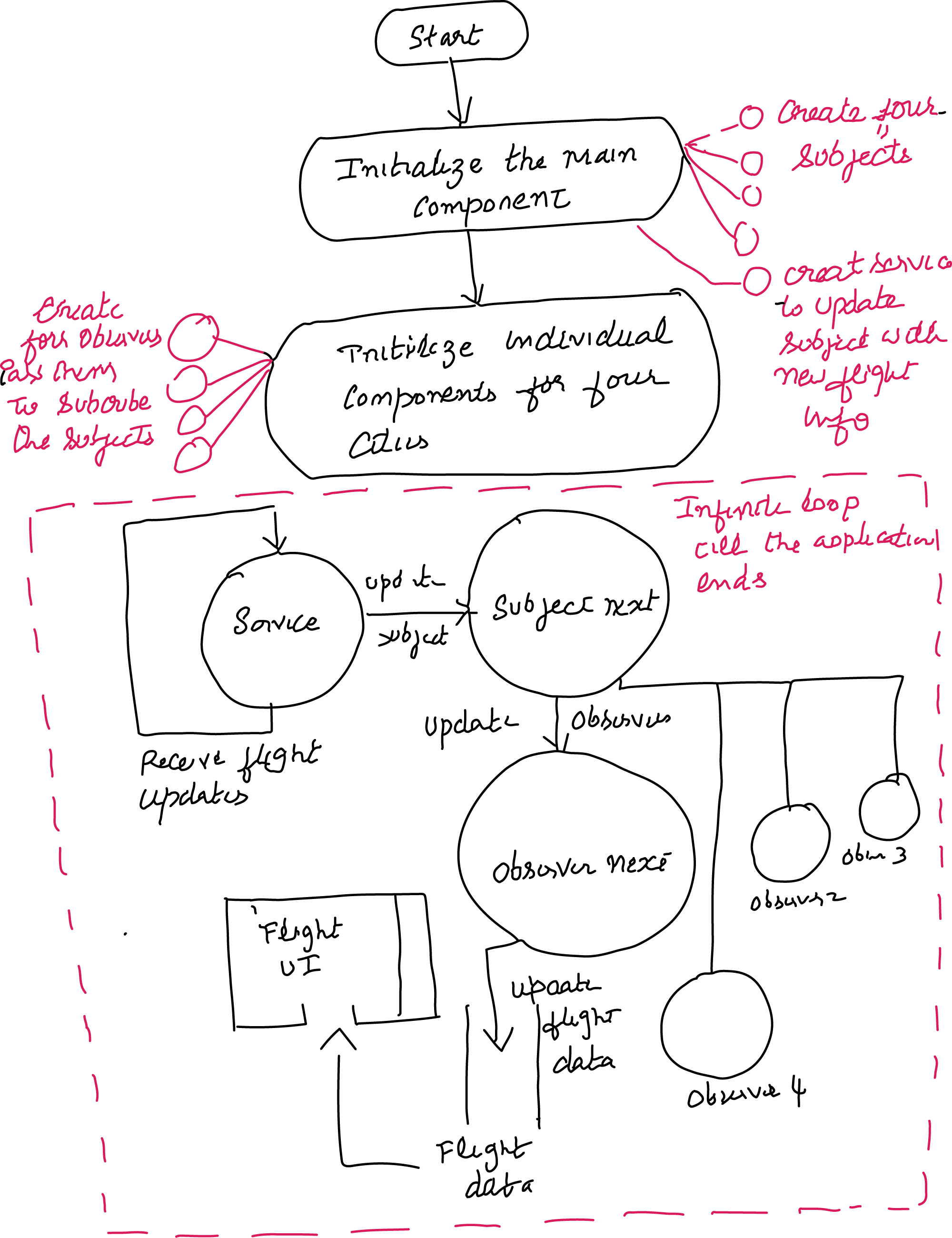
Code excerpts
Subjects created at app initiation:

Services to update subjects with latest flight information
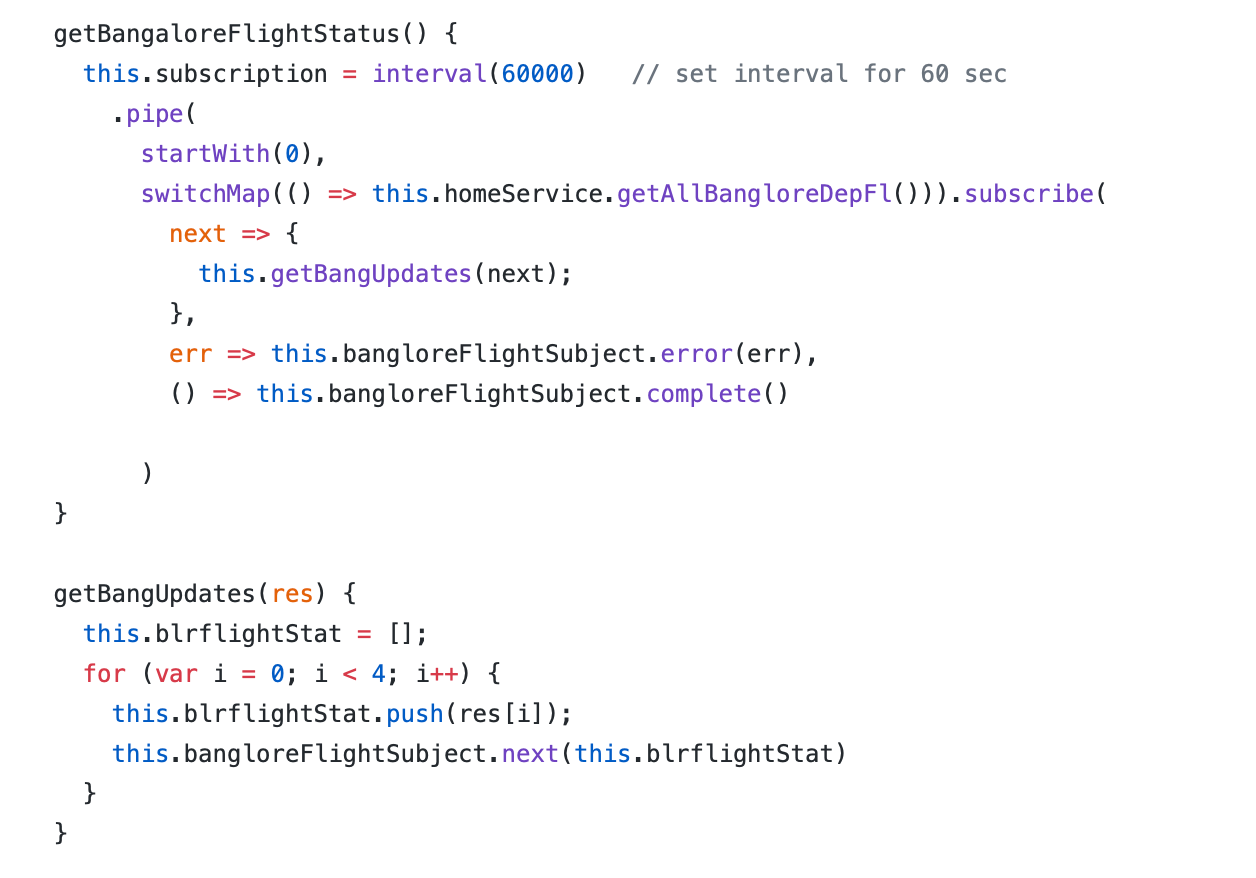
Subscribing to services in component
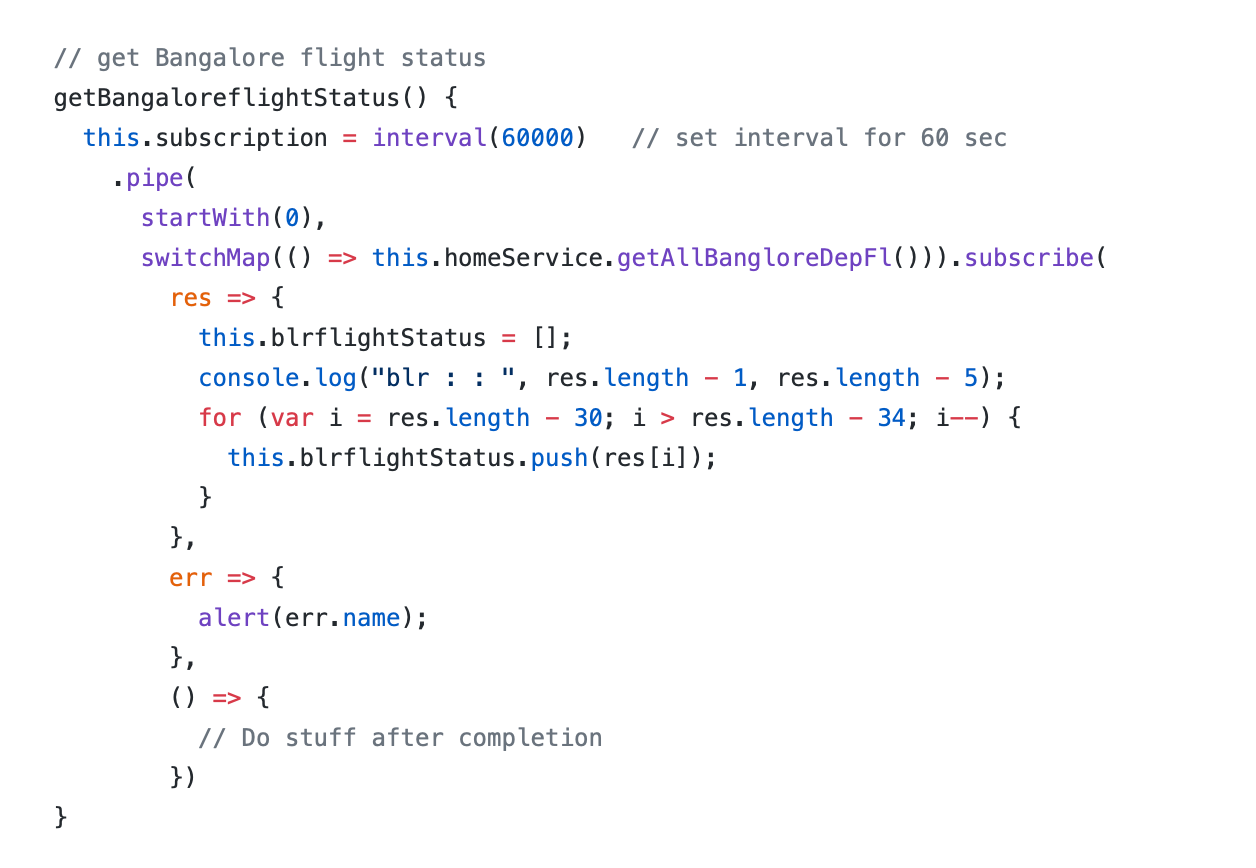
Summing it up
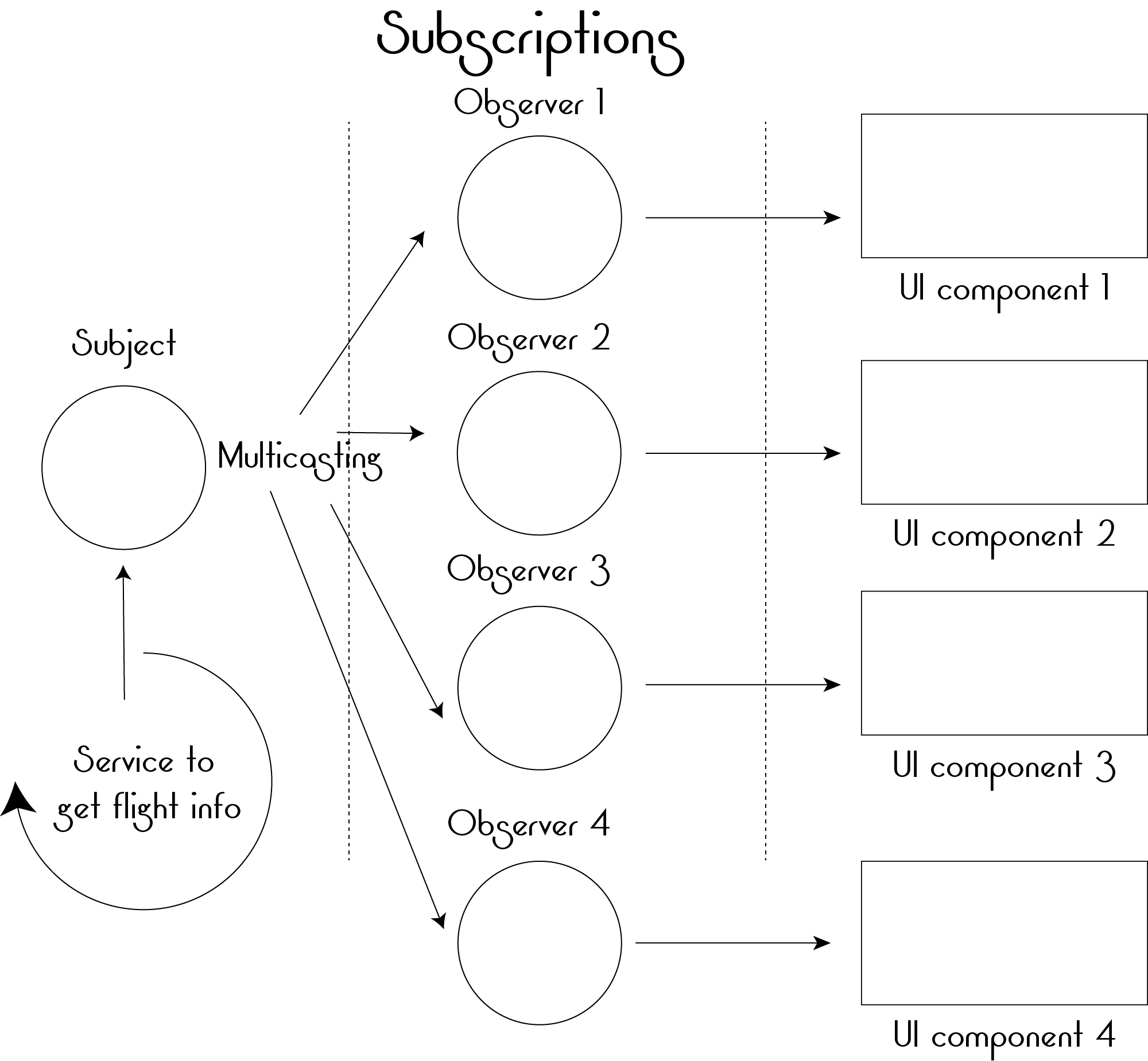
We have 3 parts in our app. All these parts execute independently.
The backend part, the middle layer part and the front end part.
The backend part comprise of the services and the subjects. The services keep running and keep updating the subjects.
The middle layer is the observers who receive the changes from the subjects and update data structure in the front end layer.
The front end layer keeps reflecting the changes onto the user interface.
We would love to discuss your solutions and requirements.
Feel free to contact us at thrive@simpragma.com